1 # Multiwii Serial Protocol Version 2
5 This page describes MSPV2 (MultiWii Serial Protocol Version 2). MSP is the remote messaging protocol used by INAV and other flight controllers such as MultiWii, CleanFlight and BetaFlight.
7 MSPV2 was introduced in INAV 1.73 for legacy commands, and is fully implemented (16bit commands) after 1.73 (i.e. 1.74 development branch and successors). An MSP API version of 2 or greater indicates MSPV2 support.
11 MSP is a request-response protocol. MSP defines the side sending the request as "MSP Master" role and the side generating a response as "MSP Slave" role.
13 A specific device may function as both "MSP Master" and "MSP Slave" or implement only one role, depending on requirements. Generally, a Groundstation software is an "MSP Master" and FC is an "MSP Slave", however in some use-cases a FC or any other device may be required to act as an "MSP Master" and "MSP Slave" concurrently.
15 ## Rationale to create MSP V2
17 The reasons for introducing a incrementally improved version of MSP include:
19 * Limited message IDs. MSP v1 provided 255 message IDs; between INAV and BetaFlight (CleanFlight), this space is almost exhausted.
20 * Limited message size. MSP v1 is limited to 255 byte message payloads. This is already a problem and can only get worse. The extant attempt to address this limitation in MSP v1 (the JUMBO frame extension) is a 'band-aid' and still suffers from the next restriction.
21 * Weak check-summing. MSP v1 uses a simple XOR checksum. This is vulnerable to simple communications errors (transposed bits). It can fail to detect common transmission corruptions.
23 MSP V2 addresses these shortcomings:
25 * 16bit message space. 65535 message IDs. For backwards compatibility, message IDs 0-255 map onto the analogous MSP v1 messages.
27 * crc8_dvb_s2 checksum algorithm. This is a single byte CRC algorithm that is much more robust than the XOR checksum in MSP v1.
29 ## MSP V2 Message structure
31 | Offset | Usage | CRC | Comment |
32 | ---- | ---- | ---- | --- |
33 | 0 | $ | | Same lead-in as V1 |
34 | 1 | X | | 'X' in place of v1 'M' |
35 | 2 | type | | '<' / '>' / '!' see [Message Types](#Message-Types) |
36 | 3 | flag | ✔ | uint8, flag, usage to be defined (set to zero) |
37 | 4 | function | ✔ |uint16 (little endian). 0 - 255 is the same function as V1 for backwards compatibility |
38 | 6 | payload size | ✔ |uint16 (little endian) payload size in bytes |
39 | 8 | payload | ✔ | n (up to 65535 bytes) payload |
40 | n+8 | checksum | | uint8, (n= payload size), crc8_dvb_s2 checksum |
42 The fields marked with a ✔ are included in the checksum calculation.
46 | Identifier | Type | Can be sent by | Must be processed by | Comment |
48 | '<' | Request | Master | Slave | |
49 | '>' | Response | Slave | Master | Only sent in response to a request |
50 | '!' | Error | Master, Slave | Master, Slave | Response to receipt of data that cannot be processed (corrupt checksum, unknown function, message type that cannot be processed) |
52 ## Encapsulation over V1
54 It is possible to encapsulate V2 messages in a V1 message in a way that is transparent to the consumer. This is implemented by setting the V1 function id to 255 and creating a payload of a V2 message without the first three header bytes.
55 Thus a V1 consumer would see a not understood message rather than a communications error. This is not encouraged; rather it is preferred that MSP consumers should implement V2.
57 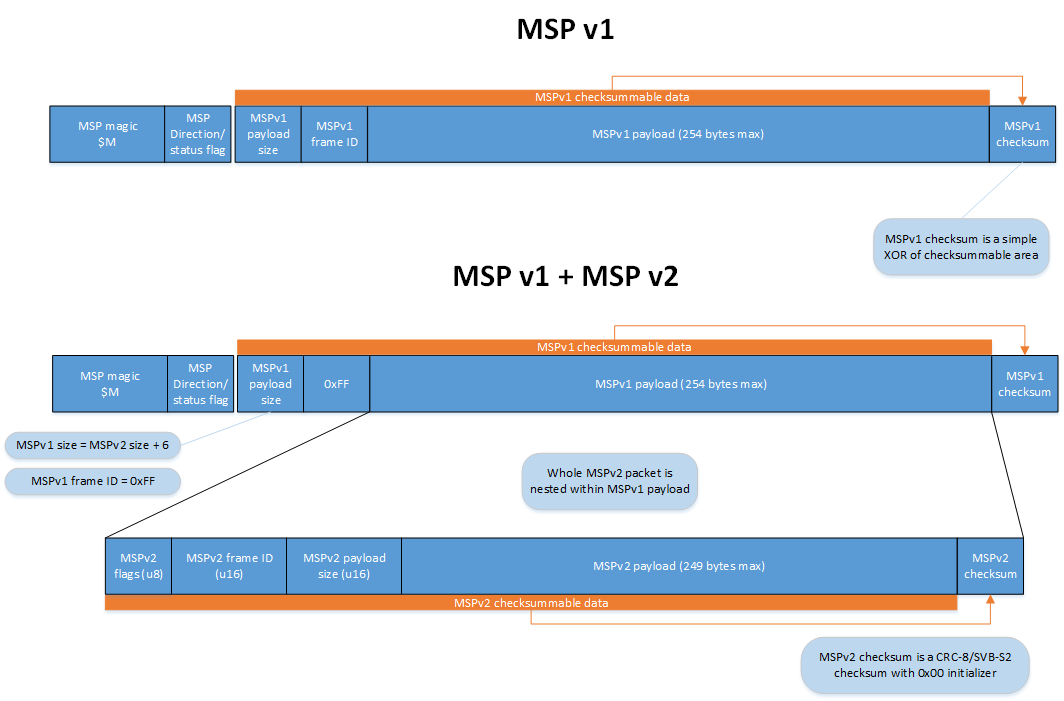
63 As MSP V2 (function id: 100, payload size: 0)
66 "$X<\x00d\x00\x00\x00\x8F"
67 24 58 3c 00 64 00 00 00 8f
71 For a mythical V2 "Hello World" message with function id 0x4242 (note: this function id is not implemented in INAV), as MSPV2 (hex bytes), 18 byte payload, flag set to 0xa5:
74 24 58 3e a5 42 42 12 00 48 65 6c 6c 6f 20 66 6c 79 69 6e 67 20 77 6f 72 6c 64 82
77 And embedded in a V1 message (hex bytes):
80 24 4d 3e 18 ff a5 42 42 12 00 48 65 6c 6c 6f 20 66 6c 79 69 6e 67 20 77 6f 72 6c 64 82 e1
82 The V2 capable CGS **mwp** reports this as:
84 2017-08-11T19:50:12+0100 MSP_CMDS_HELLO_WORLD frame (18): flag=0xa5 "Hello flying world"
86 Note: This message function is NOT implemented in the FC. It is just a (temporary) test case in mwp.
90 The checksum should be initialised to zero. The following 'C' code snippet shows the INAV implementation.
92 uint8_t crc8_dvb_s2(uint8_t crc, unsigned char a)
95 for (int ii = 0; ii < 8; ++ii) {
97 crc = (crc << 1) ^ 0xD5;
105 And **pseudo-code** usage:
107 int len; // payload size
108 uint8_t *msg = calloc(1, len+9); // allocation for message
111 // complete message content
112 uint8_t ck2 = 0; // initialise CRC
113 for (int i = 3; i < len+8; i++)
114 ck2 = crc8_dvb_s2(ck2, msg[i]); // loop over summable data
118 ## MSP v1 JUMBO Messages
120 As the MSP v1 JUMBO messages is not obviously documented elsewhere:
122 * This is a MSP v1 `$M ... ` message
123 * Set the function code as normal (0-255)
124 * Set the payload size to 255
125 * set the real real payload size as the first two bytes of the payload
126 * Then the real payload
127 * Then a MSP V1 XOR checksum as normal
129 One could embed a MSP V2 message within a MSP V1 JUMBO frame, but this is not likely to be well supported by consumers. If you need V2, please use it natively.
131 # MSP V2 Message Catalogue
133 For INAV 1.8.0, MSP V2 messages have been defined (0x4242 is a joke, not a land grab). It is hoped that a message catalogue can be cooperatively developed by FC authors to avoid the current fragmentation in MSP V1.
135 Suggested approach is to allocate blocks of MSPv2 messages to certain firmwares - use high nibble of Function ID as firmware family identifier. This will allow up to 4096 firmware-specific messages.
137 | Function ID | Usage | Supports flags | FCs implementing | Documentation Link |
138 | ----- | ---------- | ---- | ---- | ---- |
139 | 0x0000-0x00FE | Legacy | ✘ | INAV, MultiWii, BetaFlight, Cleanflight, BaseFlight | http://www.multiwii.com/wiki/index.php?title=Multiwii_Serial_Protocol |
140 | 0x1000-0x1EFF | Common messages | ✘ | INAV | |
141 | 0x1F00-0x1FFF | Sensors connected via MSP | ✘ | INAV | |
142 | 0x2000-0x2FFF | INAV-specific | ✘ | INAV | |
143 | 0x3000-0x3FFF | Betaflight-specific, Cleanflight-specific | ✘ | Betaflight, Cleanflight | |