1 # taruga [](https://www.codacy.com/gh/vrmiguel/taruga/dashboard?utm_source=github.com&utm_medium=referral&utm_content=vrmiguel/taruga&utm_campaign=Badge_Grade) [](https://www.codefactor.io/repository/github/vrmiguel/taruga)
3 Taruga is a work in progress single-header turtle graphics library written in C++11.
6 <img src="https://user-images.githubusercontent.com/36349314/95826050-236a4a80-0d08-11eb-8032-a8644704e423.gif" alt="Spiral"/>
11 ### Drawing a Sierpinski fractal
13 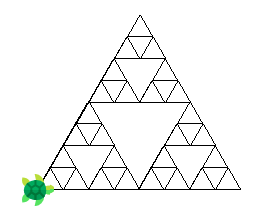
15 With the simple Turtle directives, we can build complex fractals!
17 Full source code for this example can be found [here](examples/spiral.cpp).
21 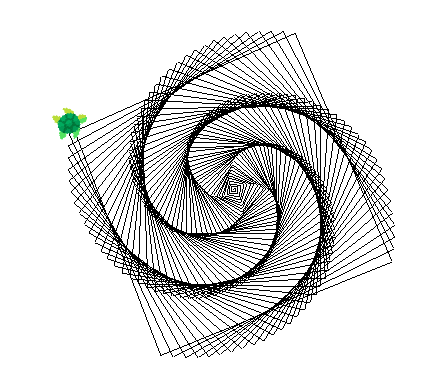
23 We can draw this spiral by simply moving forward and then turning to the right by slightly over 90°.
29 turtle.forward(size++);
30 turtle.turn_right(91);
34 Full source code for this example can be found [here](examples/spiral.cpp).
38 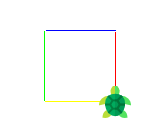
40 We can change around the color of the lines drawn by using `Turtle::set_line_color`, to which you can supply either an RGB triplet (by individual values or in an array) or a [sf::Color](https://www.sfml-dev.org/documentation/2.5.1/classsf_1_1Color.php).
43 std::vector<sf::Color> colors = {sf::Color::Red, sf::Color::Blue, sf::Color::Green, sf::Color::Yellow};
45 for(auto color : colors)
47 turtle.set_line_color(color);
53 Full source code for this example can be found [here](examples/colored_square.cpp).
55 ### Lifting the pen up, drawing regular polygons
57 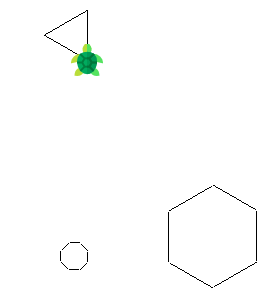
59 We can set the turtle's pen up or down. This allows us to move the turtle without having to draw the line of its path.
61 Full source code for this example can be found [here](examples/regular_polygon.cpp).
63 ### Drawing a Koch fractal
65 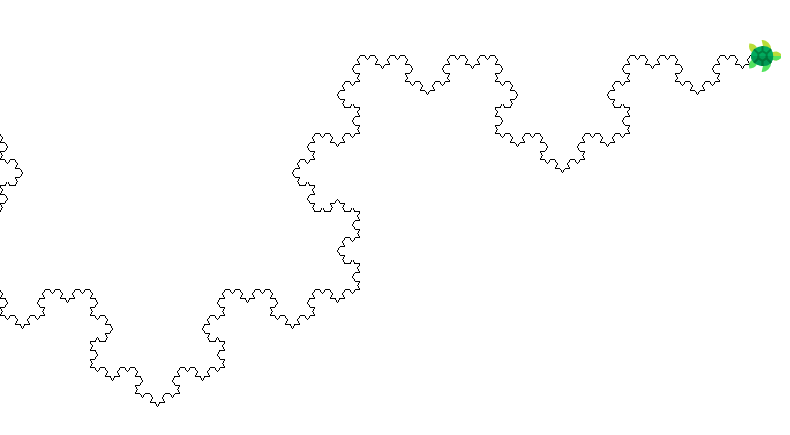
67 Full source code for this example can be found [here](examples/koch.cpp).
69 ## Dependencies and how to build
71 The only dependencies are a C++11 conformant compiler and [SMFL](https://www.sfml-dev.org/index.php), a small multimedia API with an installed size of around 10MB.
74 Taruga has been built on SFML 2.5.1 and has also been tested on SFML 2.4.1. It may work on older versions of the library, but there are no guarantees.
76 On Ubuntu-based distributions, you can install SFML with:
78 ```sudo apt install libsfml-dev```
81 ```dnf install SFML```
83 You can then build it ```g++ -O3 -std=c++11 your_program.cpp -lsfml-graphics -lsfml-window -lsfml-system```.
87 Taruga exposes a single namespace, contained within a single header file ([`taruga.hpp`](include/taruga.hpp)).
88 The public methods that `taruga::Turtle` exposes are:
91 void set_window_title(const char* new_title);
92 Turtle(); //! Default constructor. Makes a window size of 800x600 and sets the turtle to (400, 300).
93 Turtle(uint16_t width, uint16_t height); //! Makes a window size of width * height and sets the turtle (width/2, height/2).
94 explicit Turtle(const sf::Vector2f& p); //! Makes a window size of p.x * p.y and sets the turtle (p.x/2, p.y/2).
95 void save_to_image(const char *); //! Saves the current window view to an image with the given filename
96 void set_line_color(sf::Color); //! Sets the new color of the line the turtle will draw.
97 void set_line_color(u8, u8, u8); //! Sets the new color of the line the turtle will draw.
98 void set_line_color(u8[3]); //! Sets the new color of the line the turtle will draw.
99 void pen_up(); //! Sets the pen up so lines don't get drawn
100 void pen_down(); //! Sets the pen down so that the turtle draws a line wherever it walks
101 void set_icon(Icon); //! Allows to switch around between the two built-in icons: turtle or straight arrow.
102 void set_icon(sf::Texture); //! Allows for any image to be used as an icon. Do notice that Taruga won't scale the texture. If needed, use the Turtle::scale method.
103 void scale(float, float); //! Scales the turtle sprite
104 void forward(float units); //! Walk forward the given amount of units.
105 void backwards(float units); //! Walk backwards the given amount of units. The same as using forward() with a negative parameter.
106 void turn_right(float ang); //! Turns right by the specified amount of degrees.
107 void set_walking_speed(float); //! Sets a new walking speed
108 void set_rotation_speed(float); //! Sets a new rotation speed
109 void push_state(); //! Saves the current state in a stack
110 void pop_state(); //! Returns the Turtle's state to the top of the state stack
111 void turn_left(float ang); //! Turns left by the specified amount of degrees.
112 void act(); //! Start moving the turtle. Will deplete the actions queue.
117 The code contained in this repo is [MIT](https://opensource.org/licenses/MIT)-licensed.
119 Turtle icon made by Freepik, available under the [Flaticon](https://www.flaticon.com/) license.
121 SFML is [zlib/libpng](https://opensource.org/licenses/Zlib)-licensed.